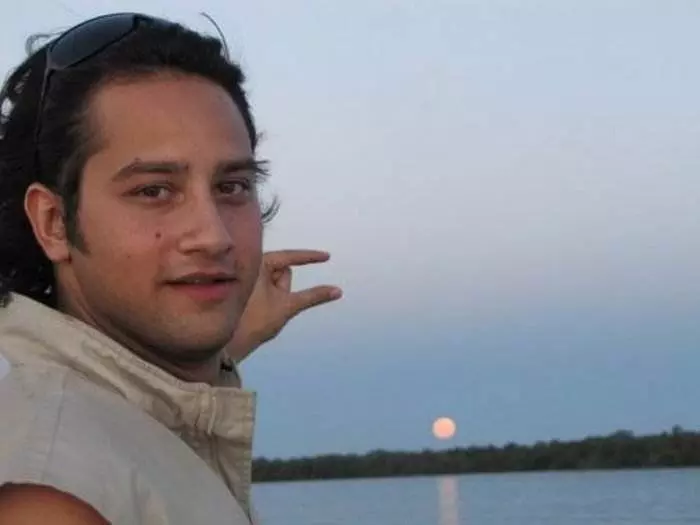
Learn to identify them. Develop habits to avoid them.
The goal of this article is not to stump newbies for common mistakes, but to teach them to identify and avoid them. The enumeration order is random.
From translator
Sometimes it is difficult to explain with simple words, seemingly trivial things: why use git, what is the encapsulation feature, why write tests, how to plan your code, refactor someone else's, etc. It seemed to me that in this article the important "humanitarian" aspects of programming are compactly collected. Something like a moral code, a guide and a motivator in making decisions related to writing code.
No matter how ridiculous it may sound, I have been working on this text since mid-March, trying to find suitable wording and make it easier to read. A couple of days he fought with the habra editor. Therefore, if you find shortcomings, I ask you not to blame me for negligence, but to inform me, I will correct them immediately. I thought to decorate the article with pictures, but I decided that it would only inflate it to completely indecent sizes. Enjoy reading.
1) Programming without planning2) Over planning3) Underestimating the importance of code quality4) Grasp the first decision5) Do not retreat6) Do not google7) Do not use encapsulation8) Planning for the unknown9) Use of inappropriate data structures10) worsen the code11) Commenting on obvious things.12) Do not write tests13) To think if something works, then it is properly done14) Do not question the existing code.15) Obsession with best practices16) Performance Obsession17) Do not focus on the end user18) Do not pick the right tools.19) Misunderstanding that problems with code cause problems with data20) The invention of the wheel21) Incorrect attitude to code inspection (code review)22) Not using version control systems23) Shared State Abuse24) Incorrect attitude to errors25) Do not rest1) Programming without planning
Quality content is not created “on the knee”, but requires thorough work. Program code is no exception.
A good code must go through the following stages:
The idea. Study. Planning. Writing Check. Change.Each of these points should be given enough effort.
Newbies tend to code without preliminary thought. This might work on small standalone applications. But the big ones can turn into tragedy.
Before you say something, you think about the words so that later you are not ashamed. Similarly, avoid an ill-conceived code for which one day it will be a shame. Both words and code are a representation of your thoughts.
“If you're angry, count to 10 before you speak.” If very angry, then up to 100 ". (Thomas Jefferson)
For our case, this can be rephrased as:
“When checking the code, count to 10 before rewriting 1 line. If there are no tests for this code, then up to 100 ”.
Programming is a 90% study of existing code and its modification through small, easily tested portions that fit into the overall system. Code writing itself is only 10% of the programmer's work.
Programming is not just writing lines of code, but creativity based on logic that needs to be developed in oneself.
2) Over planning
Planning before diving into writing code is a good thing. But even good things can hurt you if you overdo it. Even water can be poisoned if you drink too much of it.
Do not look for the perfect plan. This does not exist in the programming world. Look for a good enough plan to start. Any plan will change, but it will force you to adhere to the structure in the code, which will facilitate your future work.
Linear planning of the entire program “from A to Z” (by the waterfall method) is not suitable for most software products. Development implies feedback and you will constantly remove and add functionality, which cannot be taken into account in the “waterfall planning”. The following items should be planned. And each new one should be included in the plan only after a flexible adaptation to reality (Agile).
Planning must be approached very responsibly, because its lack and excess can harm the quality of the code. And the quality of the code in any case can not risk it.
3) Underestimating the importance of code quality
If while writing code you can focus on only one thing, then it should be readable. The incomprehensible unreadable code is not just garbage, but garbage that cannot be recycled.
Look at the program as parts communicating through code. Bad code is bad communication.
“Write your code as if it would be accompanied by an aggressive psychopath, who knows where you live.” (John Woods)
Even the “little things” are important. If you randomly use capital letters and indents, then you need to select a programmer's license.
tHIS is
WAY MORE important
than
you think
. 80 . (ESLint, Prettier js).
. , . 10 – .
. . .
, , . , , , .
“ : ”. ( )
. - 12, :
const monthsInYear = 12;
. , .
. . , . . – , .
“ , , ”. ( )
. , , . , .
.
4)
, , , . , , , . , , .
, , . , , .
“ : 1) , , , ; 2) , ”. ( )
5)
– . , . “ ” , .
. – . , . Git , .
, . .
6)
, , - . , .
. , , . , , .
– . , .
, :
“, , – ”.
7)
, . .
, . , . , . , .
. ,
,
,
.
. – . , – .
, , . , “ ”. .
,
(High Cohesion and Low Coupling). , , – .
8)
, : “ …” , . .
, . , - .
, . , , .
“ — ”. ( )
9)
. – . .
, , .
: “ !”. .
?
– .
:
[{id: 1, title: "entry1"}, {id: 2, title:"entry2"}, .... ]
:
{ 1: {id: 1, title: "entry1"}, 2: {id: 2, title:"entry2"}, ....}
, , . , . , push, pop, shift, unshift, , .
, , . , , .
?
, . , 2 .
. , .
10)

, . - . . . . — , -. , , , , . , .
, :
, , . , , . , .
, :
, , : “ ?” “”.
if , , . – : ?
if:
function isOdd(number) {
if (number % 2 === 1) {
return true;
} else {
return false;
}
}
if:
function isOdd(number) {
return (number % 2 === 1);
};
11)
. , .
, :
// This function sums only odd numbers in an array
const sum = (val) => {
return val.reduce((a, b) => {
if (b % 2 === 1) { // If the current number is odd
a+=b; // Add current number to accumulator
}
return a; // The accumulator
}, 0);
};
:
const sumOddValues = (array) => {
return array.reduce((accumulator, currentNumber) => {
if (isOdd(currentNumber)) {
return accumulator + currentNumber;
}
return accumulator;
}, 0);
};
. : “ ”, “ ”. , :
// create a variable and initialize it to 0
let sum = 0;
// Loop over array
array.forEach(
// For each number in the array
(number) => {
// Add the current number to the sum variable
sum += number;
}
);
, . — .
12)
, , – .
, . -, - . , . , , . , - , , .
, , - . .
, . (test-driven development, TDD) . , , .
TDD , , , .
13) , - ,
, . ?
const sumOddValues = (array) => {
return array.reduce((accumulator, currentNumber) => {
if (currentNumber % 2 === 1) {
return accumulator + currentNumber;
}
return accumulator;
});
};
console.assert(
sumOddValues([1, 2, 3, 4, 5]) === 9
);
. . ?
, . .
1
. , ? .
TypeError: Cannot read property 'reduce' of undefined.
:
.
.
, . , :
TypeError: Cannot execute function for empty list.
, 0? , - , .
2
. , ? :
sumOddValues(42);
TypeError: array.reduce is not a function
, array.reduce — . array (), ( 42), . , 42.reduce — . :
: 42 - , .
1 2 , . , . , , ?
sumOddValues([1, 2, 3, 4, 5, -13]) // => still 9
-13 ? ? ? “ ”? . , , , , , , .
“ . ” — , .
3
. . .
sumOddValues([2, 1, 3, 4, 5]) // => 11
2 , . , reduce initialValue, . . , .
14)
- , , . , , .
, , , .
, . .
:
, — , . . .
git blame, .
, , . , . .
15)
“ ” , , « ».
“ ” . .
, “ ” . , . “ ”, , .
-, - , - , - “ ”. , , , .
16)
“ – ( )”. , 1974
, .
“ ”, . , , .
, , . , Node.js .
.
17)
, ? , . ? . ?
. , , . , . , .
18)
. - , . . , , 5.0.
, – .
, “” . .
, . .
. , . , .
19) ,
— . – , .
. , . , . , .
, , , .
? : , , (). , .
.
NOT NULL , . , .
UNIQUE . .
CHECK , , 0 100.
PRIMARY KEY . .
FOREIGN KEY , .
–
. , , , , .
20)
. . .
, , , , . , , , . .
- . . . “ ” . (open source), , , .
, , . - .
shuffle lodash, , lodash.
21) (code review)
, . , .
. . . . .
, – , – .
-. , , , . , .
22) (Git)
/, Git.
, . — . . , , .
, . . , , .
, . , . .
– . , . , , , , .
, , , .
bisect, , .
, , :
- (staging changes)
- (patching selectively)
- (resetting)
- (stashing)
- (amending)
- (applying)
- (diffing)
- (reversing)
, , . Git , .
23) (shared state)
.
. . , , . , , . , . .
, ( ). (Race conditions). , . . . .
24)
– . , . , – .
– . , , , .
– . .
25)
. . -, . , , , . .