Hi, Habr! Once I needed to create a 3D model of the bottom,
more in this article . Today I want to talk about how to create 3D models of Python 3. There are many ways to do this: blender python api, Vpython ... But I want to tell you how to make models using only Python.
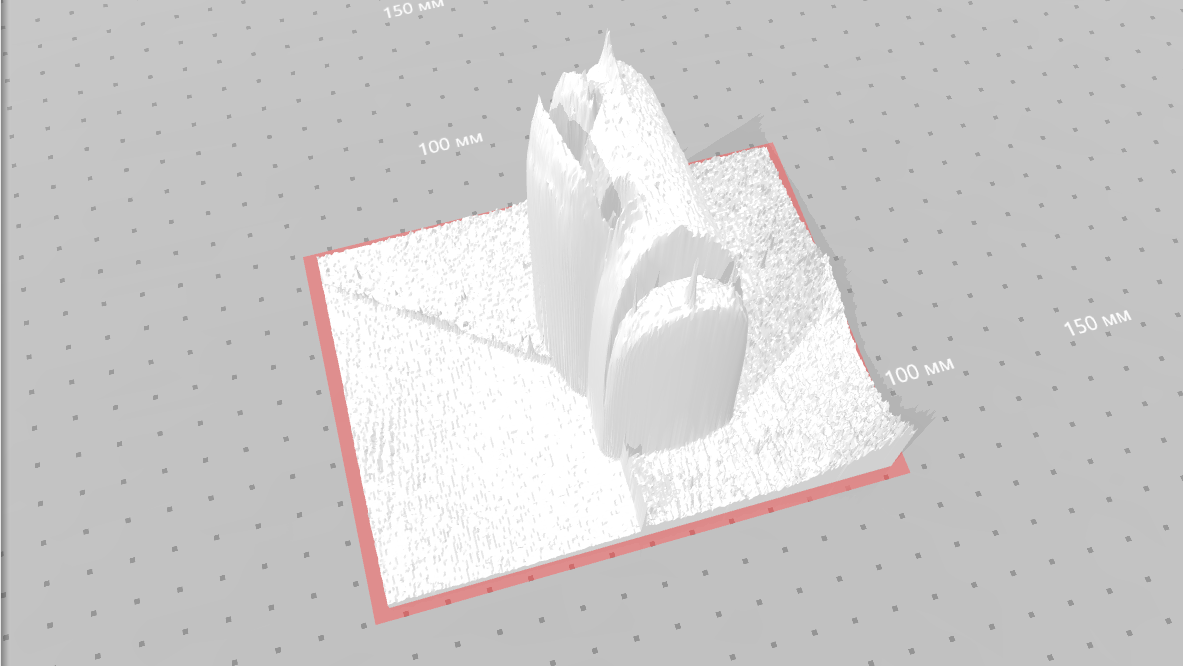
→
Link to GithubSTL
To do this, you need to understand how the stl format (the popular 3D file format) works.
The whole model in this format consists of many triangles, so the file consists of 3-dimensional coordinates of their vertices.
STL filesolid
facet normal 0 0 0
outer loop
vertex 0 0 0
vertex 1 0 0
vertex 1 1 0
endloop
endfacet
facet normal 0 0 0
outer loop
vertex 1 1 0
vertex 0 0 0
vertex 0 1 0
endloop
endfacet
endsolid
Example
I would like to show how to implement the creation of a 3D model according to the brightness of the pixels in the photo. I took this photo below.

The image is processed (slightly blurred so that there are no sharp changes in brightness) using the opencv library.
import cv2 import numpy as np cd_1=['0', '0', '0']
Bottom, a function that takes 3 arrays with coordinates of triangle vertices and writes 1 triangular face to the file
def face_file_stl(cd_1, cd_2, cd_3): op_stl.write("facet normal 0 0 0") op_stl.write("outer loop") op_stl.write("vertex " + " ".join(cd_1))
Now the most important thing is to create the correct coordinates of the vertices of the triangles of which the 3D model consists.

The part of the code that creates the coordinates.
for i in range(size.shape[1]):
Created 3D modelActually, this is all that I wanted to write before the next article.
All code import cv2 cd_1=['0', '0', '0']